07 Dec 2014
Day 18: Php Oops With MySql (Update and Delete Records)
Continue to the day 17, now we are going to edit, update and delete records with OOPS
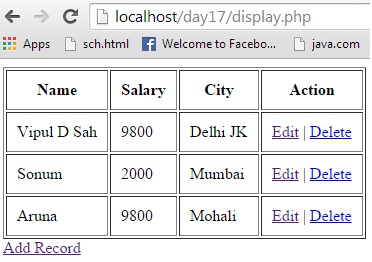
Program 52: edit, update and delete code in “db1.php”
<?php
class emp {
var $con = “”;
var $id; var $name;
var $salary;
var $city;
function __construct() {
$this->con = mysql_connect(‘localhost’,’root’,”);
mysql_select_db(‘mydb’,$this->con);
}
function display() {
$query = “select * from tbemp”; $data = mysql_query($query); return $data;
}
function save() {
$query = “insert into tbemp (name,salary,city) values (‘$this->name’,’$this->salary’,’$this->city’)”; if(mysql_query($query)) {
return true;
} else {
return false;
}
}
function getbyid() {
$query = “select * from tbemp where id = $this->id”;
$data = mysql_query($query);
$rec = mysql_fetch_object($data);
return $rec;
}
function update() {
$query = “update tbemp set name=’$this->name’, salary=’$this->salary’, city=’$this->city’ where id = $this->id”;
if(mysql_query($query)) {
return true;
} else {
return false;
}
}
function delete() {
$query = “delete from tbemp where id = $this->id”;
mysql_query($query);
header(“location:display.php”);
}
} ? >
class emp {
var $con = “”;
var $id; var $name;
var $salary;
var $city;
function __construct() {
$this->con = mysql_connect(‘localhost’,’root’,”);
mysql_select_db(‘mydb’,$this->con);
}
function display() {
$query = “select * from tbemp”; $data = mysql_query($query); return $data;
}
function save() {
$query = “insert into tbemp (name,salary,city) values (‘$this->name’,’$this->salary’,’$this->city’)”; if(mysql_query($query)) {
return true;
} else {
return false;
}
}
function getbyid() {
$query = “select * from tbemp where id = $this->id”;
$data = mysql_query($query);
$rec = mysql_fetch_object($data);
return $rec;
}
function update() {
$query = “update tbemp set name=’$this->name’, salary=’$this->salary’, city=’$this->city’ where id = $this->id”;
if(mysql_query($query)) {
return true;
} else {
return false;
}
}
function delete() {
$query = “delete from tbemp where id = $this->id”;
mysql_query($query);
header(“location:display.php”);
}
} ? >
Program 53: place a link of edit and delete in “display.php”
<?php
require_once “db1.php”;
$empObj = new emp();
$data = $empObj->display();
?>
<html>
<head>
<title>Display Records</title>
</head>
<table cellpadding=”10″ border=”1″>
<tr> <th>Name</th><th>Salary</th><th>City</th> <th>Action</th>
</tr>
<?php
while($rec = mysql_fetch_object($data)) { ?>
<tr> <td><?php echo $rec->name; ?></td>
<td><?php echo $rec->salary; ?></td>
<td><?php echo $rec->city; ?></td>
<td><a href=”edit.php?id=<?php echo $rec->id; ?>”>Edit</a> | <a onClick=”return confirm(‘Sure to Delete!’)” href=”delete.php?id=<?php echo $rec->id; ?>”>Delete</a></td>
</tr> <?php } ?>
</table>
<a href=”save.php”>Add Record</a>
</html>
require_once “db1.php”;
$empObj = new emp();
$data = $empObj->display();
?>
<html>
<head>
<title>Display Records</title>
</head>
<table cellpadding=”10″ border=”1″>
<tr> <th>Name</th><th>Salary</th><th>City</th> <th>Action</th>
</tr>
<?php
while($rec = mysql_fetch_object($data)) { ?>
<tr> <td><?php echo $rec->name; ?></td>
<td><?php echo $rec->salary; ?></td>
<td><?php echo $rec->city; ?></td>
<td><a href=”edit.php?id=<?php echo $rec->id; ?>”>Edit</a> | <a onClick=”return confirm(‘Sure to Delete!’)” href=”delete.php?id=<?php echo $rec->id; ?>”>Delete</a></td>
</tr> <?php } ?>
</table>
<a href=”save.php”>Add Record</a>
</html>
Program 54: code to view edited record in “edit.php”
<?php require_once “db1.php”;
$empObj = new emp();
$msg = “”;
$id = isset($_GET[‘id’]) ? $_GET[‘id’] : 0;
if($id > 0) {
$empObj->id = $id; $empData = $empObj->getbyid();
} else {
header(“location:display.php”);
}
if(isset($_REQUEST[‘btnSubmit’])) {
if(trim($_REQUEST[‘txtName’])!=””) {
$empObj->name = $_REQUEST[‘txtName’];
} else {
$msg = “*Name Required”;
}
if(trim($_REQUEST[‘txtSalary’])!=””) {
$empObj->salary = $_REQUEST[‘txtSalary’];
} else {
$msg.= “<br/>*Salary Required”;
}
if(trim($_REQUEST[‘txtCity’])!=””) {
$empObj->city = $_REQUEST[‘txtCity’];
} else {
$msg.= “<br/>*City Required”;
} if($msg == “”) {
$result = $empObj->update();
if($result) {
header(“location:display.php”);
} else {
$msg = “Unable To Update!”;
}
}
} ?>
<html>
<head>
<title>Edit Record</title>
</head>
<div id=”msg” style=”color:red”>
<?php echo $msg; ?>
</div>
<form method=”POST”>
<table cellspacing=”10″>
<tr> <th>Name* : </th> <td>
<input type=”text” name=”txtName” id=”txtName” value=”<?php echo isset($empData->name) ? $empData->name : “”; ?>”/> </td>
</tr>
<tr> <th>Salary* : </th> <td> <input type=”text” name=”txtSalary” id=”txtSalary” value=”<?php echo isset($empData->salary) ? $empData->salary : “”; ?>”/>
</td> </tr>
<tr> <th>City* : </th>
<td> <input type=”text” name=”txtCity” id=”txtCity” value=”<?php echo isset($empData->city) ? $empData->city : “”; ?>”/> </td> </tr>
<tr> <td></td><td><input type=”submit” value=”Update” name=”btnSubmit” id=”btnSubmit”/></td> </tr> </table>
</form> <a href=”display.php”>Back</a>
</html>
$empObj = new emp();
$msg = “”;
$id = isset($_GET[‘id’]) ? $_GET[‘id’] : 0;
if($id > 0) {
$empObj->id = $id; $empData = $empObj->getbyid();
} else {
header(“location:display.php”);
}
if(isset($_REQUEST[‘btnSubmit’])) {
if(trim($_REQUEST[‘txtName’])!=””) {
$empObj->name = $_REQUEST[‘txtName’];
} else {
$msg = “*Name Required”;
}
if(trim($_REQUEST[‘txtSalary’])!=””) {
$empObj->salary = $_REQUEST[‘txtSalary’];
} else {
$msg.= “<br/>*Salary Required”;
}
if(trim($_REQUEST[‘txtCity’])!=””) {
$empObj->city = $_REQUEST[‘txtCity’];
} else {
$msg.= “<br/>*City Required”;
} if($msg == “”) {
$result = $empObj->update();
if($result) {
header(“location:display.php”);
} else {
$msg = “Unable To Update!”;
}
}
} ?>
<html>
<head>
<title>Edit Record</title>
</head>
<div id=”msg” style=”color:red”>
<?php echo $msg; ?>
</div>
<form method=”POST”>
<table cellspacing=”10″>
<tr> <th>Name* : </th> <td>
<input type=”text” name=”txtName” id=”txtName” value=”<?php echo isset($empData->name) ? $empData->name : “”; ?>”/> </td>
</tr>
<tr> <th>Salary* : </th> <td> <input type=”text” name=”txtSalary” id=”txtSalary” value=”<?php echo isset($empData->salary) ? $empData->salary : “”; ?>”/>
</td> </tr>
<tr> <th>City* : </th>
<td> <input type=”text” name=”txtCity” id=”txtCity” value=”<?php echo isset($empData->city) ? $empData->city : “”; ?>”/> </td> </tr>
<tr> <td></td><td><input type=”submit” value=”Update” name=”btnSubmit” id=”btnSubmit”/></td> </tr> </table>
</form> <a href=”display.php”>Back</a>
</html>
Program 55: code to delete the record in “delete.php”
<?php require_once “db1.php”;
$empObj = new emp();
$msg = “”;
$id = isset($_GET[‘id’]) ? $_GET[‘id’] : 0;
if($id > 0) {
$empObj->id = $id;
$empData = $empObj->delete();
} else {
header(“location:display.php”);
} ?>
$empObj = new emp();
$msg = “”;
$id = isset($_GET[‘id’]) ? $_GET[‘id’] : 0;
if($id > 0) {
$empObj->id = $id;
$empData = $empObj->delete();
} else {
header(“location:display.php”);
} ?>
Leave a Reply
You must be logged in to post a comment.
No Responses