JavaScript Archive
08 Jan 2019
JavaScript – Function
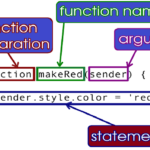
A function is a group of code designed to perform a particular task and can executed when they are called. It helps to write a modular codes. Functions allow a programmer to divide a big program into a number of small and manageable functions. We can call functions again and again when needed, but they
17 Dec 2018
JavaScript for in loop
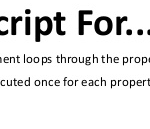
The for-in loop is used to loop through an object’s properties. I have not posted any article on Objects yet, so you may not feel comfortable with this loop. But once you got the knowledge of objects in JavaScript, you will find this loop very useful with JavaScript Objects. Syntax for (variable_name in object) {
05 Dec 2018
JavaScript For Loop
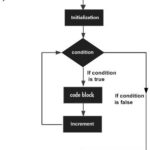
For loop provides a concise way of writing the loop structure and it’s most compact form of looping. The for loop requires following three parts: Initializer: Initialize our counter variable to start with Condition: specify a condition that must evaluate to true for next iteration, otherwise the control will come out of the loop Iteration:
03 Dec 2018
JavaScript – While Loop
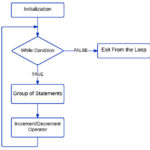
The while Loop While writing a program, when we encounter a situation where we need to perform repetitive action, in that we would need to write loop statements to reduce the number of code lines. JavaScript includes while loop to execute code repeatedly till it satisfies the given condition. Flow Chart Syntax while (condition expression){
30 Nov 2018
JavaScript – Switch Case
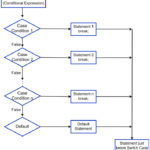
In our previous article we learned about decision making statement if-else. switch case statement is also used for decision making purposes. It’s a multi-way branch statement and in some cases switch case statement is more convenient over if-else statements, like when we want to test a variable for hundred different values and according to those
27 Nov 2018
JavaScript if-else Statement
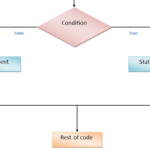
If-else Statement is one of the conditional statement, that is used to decide the flow of execution on the basic of condition, If a condition is true, you can perform one action and if the condition is false, you can perform another action. However ‘else’ is optional with ‘if’ statement, if we want to perform
12 Oct 2018
Filter Html table with JavaScript
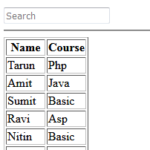
In this post, we will learn how to filter records from table with the help of JavaScript. Filter / Search in table with JavaScripit: <html> <head>Search in table</head> <body> <h2>My Students</h2> <input type=”text” id=”txtSearch” onkeyup=”search()” placeholder=”Search” title=”Type Name Or Course”> <hr/> <table id=”studentRecords” border=”1″> <tr> <th style=”width:50%;”>Name</th> <th style=”width:50%;”>Course</th> </tr> <tr> <td>Tarun</td> <td>Php</td> </tr> <tr>
27 Jul 2018
JavaScript Operators
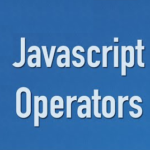
JavaScript operators are symbols that used to perform operations on operands, like in expression ‘8 + 2 = 10’, here 8 and 2 are operands and ‘+’ is operator. Types of operators in JavaScript: Assignment Operators Arithmetic Operators Comparison Operators Logical Operators Conditional (or ternary) Operators Let’s discuss all the above operators one by one
18 Jul 2018
JavaScript Variables
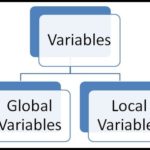
Variables are used as a containers to store the data values. We can place data into these containers and then refer to the data simply by naming the container. JavaScript allows us to work with 3 primitive data types: Numbers (Example 185, 9.5, etc.) Strings (Example ‘This is string example’) Boolean (true or false) Before
07 Jan 2018
JavaScript – Placement
Where to place JavaScript JavaScript code must be placed between <script></script> tags in html. It can be placed in the <body>, or in the <head> section of HTML page, or in both. Example: <html> <head> <script> alert(“Hello World”); </script> <head> <body></body> </html> <html> <head> <title>Internal Script</title> <script>alert("Hello World");</script> </head> </html> Functions in JavaScript Function is